Read JSON file in JavaScript
Read JSON(JavaScript Object Notation) file using JavaScript. JSON stands for JavaScript Object Notation. It means that a script (executable) file which is made of text in a programming language, is used to store and transfer the data. The data can be easily processed if it is in JSON format. The JSON file which we import can be either from the local server or a web API. There are basically three methods used to perform this task.
- JavaScript fetch() Method: Request data from a server, this request can be of any type of API that returns the data in JSON or XML.
- NodeJS require() Function: Invokes that require() function with a file path as the function’s only argument, Node goes through the following sequence of steps:
- Resolving and Loading
- Wrapping
- Execution
- Returning Exports
- Caching
- JavaScript ES6 import Module: You can import a variable using the import keyword. You can specify one of all the members that you want to import from a JavaScript file.
Note: JavaScript supports JSON internally so it does not require additional modules to import and display the JSON. We just have to import the JSON file and can easily use it directly to perform manipulations on it.
We will use the JSON file below for all approaches implementations
sample.json
{ "users":[ { "site":"GeeksForGeeks", "user": "Shobhit" } ] }
Approach(Using fetch method):
- Create a JSON file and add data to it
- Open the JavaScript file
- In the fetch, method pass the address of the file
- Use the .json method to parse the document
- Display the content on the console.
Example: In this example, we will implement the above approach.
- index.html: This file contains the script tag to run the program on the browser
- script.js: Here the fetch method is implemented which receives the JSON file and displays it on the console.
HTML
|
Javascript
|
Output:
Approach(Using require module):
- Create the JSON file as specified in the previous approach
- Create a script.js and use the require method of the node to import the JSON file
- Print the data on the console
Note: Instead of running the program on the browser we will run it on the console using Node. Make sure you have at least Node version 17.0.
script.js
Javascript
|
Steps to run the application:
Open the current folder in the terminal and type the following command.
node script.js
Output:
{ users: [ { site: 'GeeksForGeeks', user: 'Shobhit' } ] }
Approach(Using import module):
- Create the JSON file as described in the previous examples.
- Crate script.js file and import the JSON file
Javascript
|
Steps to run the application:
Open the current folder in the terminal and type the following command.
node script.js
Output:
{ users: [ { site: 'GeeksForGeeks', user: 'Shobhit' } ] }
Read JSON(JavaScript Object Notation) file using JavaScript. JSON stands for JavaScript Object Notation. It means that a script (executable) file which is made of text in a programming language, is used to store and transfer the data. The data can be easily processed if it is in JSON format. The JSON file which we import can be either from the local server or a web API. There are basically three methods used to perform this task.
- JavaScript fetch() Method: Request data from a server, this request can be of any type of API that returns the data in JSON or XML.
- NodeJS require() Function: Invokes that require() function with a file path as the function’s only argument, Node goes through the following sequence of steps:
- Resolving and Loading
- Wrapping
- Execution
- Returning Exports
- Caching
- JavaScript ES6 import Module: You can import a variable using the import keyword. You can specify one of all the members that you want to import from a JavaScript file.
Note: JavaScript supports JSON internally so it does not require additional modules to import and display the JSON. We just have to import the JSON file and can easily use it directly to perform manipulations on it.
We will use the JSON file below for all approaches implementations
sample.json
{ "users":[ { "site":"GeeksForGeeks", "user": "Shobhit" } ] }
Approach(Using fetch method):
- Create a JSON file and add data to it
- Open the JavaScript file
- In the fetch, method pass the address of the file
- Use the .json method to parse the document
- Display the content on the console.
Example: In this example, we will implement the above approach.
- index.html: This file contains the script tag to run the program on the browser
- script.js: Here the fetch method is implemented which receives the JSON file and displays it on the console.
HTML
|
Javascript
|
Output:
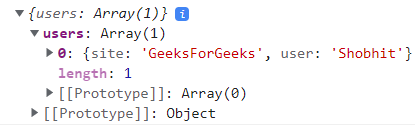
Approach(Using require module):
- Create the JSON file as specified in the previous approach
- Create a script.js and use the require method of the node to import the JSON file
- Print the data on the console
Note: Instead of running the program on the browser we will run it on the console using Node. Make sure you have at least Node version 17.0.
script.js
Javascript
|
Steps to run the application:
Open the current folder in the terminal and type the following command.
node script.js
Output:
{ users: [ { site: 'GeeksForGeeks', user: 'Shobhit' } ] }
Approach(Using import module):
- Create the JSON file as described in the previous examples.
- Crate script.js file and import the JSON file
Javascript
|
Steps to run the application:
Open the current folder in the terminal and type the following command.
node script.js
Output:
{ users: [ { site: 'GeeksForGeeks', user: 'Shobhit' } ] }