Using the useRef hook to change an element’s style in React
In this article, we will learn how to change an element’s style using the useRef hook. UseRef() allows us to create a reference to a DOM element and keep track of variables without causing re-renders.
The useRef has a lot of importance in development, one of which is accessing the DOM directly. The useRef hook enables us to handle DOM manipulations. We can directly access DOM elements by adding a ref attribute to an element. By accessing the DOM, we can even manipulate the styles of an element. We can use the style property to set styles on the DOM element.
Syntax:
const refContainer = useRef(initialValue);
To use a ref to change an element’s style in React:
- We will import the useRef hook to access the DOM element we wish to style
- Then, declare a ref and pass it to the DOM element as the ref attribute.
- useRef returns a reference object having a single property, i.e., current.
- React puts a reference to the DOM element into the ref current, which we can access through an event handler.
- We will access the element via the current property on the ref.
- Finally, we will update the element’s style through ref.current.style.
Let us understand this concept through the following example.
Approach: We will create a div element with some text. Then we will use the useRef hook to change the style of this div. We will create a button that will enable dark mode, i.e., change the div’s background to black. Below is the step-by-step implementation.
Step 1: Make a project directory, head over to the terminal, and create a react app named “gfg-card” using the following command:
npx create-react-app gfg-card
Step 2: After the gfg-card app is created, switch to the new folder gfg-card using the following command:
cd gfg-card
Step 3: We will modify the folder and keep the files we need for this project. Make sure your file structure looks like this.
Project Structure: It will look like the following.
Final Directory Structure
Step 5: Add code to your index.html file. Include the following code in your index.html file, located in the public folder of your project directory.
File name: index.html
HTML
|
Step 6: Now change the style of the div element by using the useRef hook. In the App component, create a div element and a button to enable the dark mode for the div. We will add an event handler to the button. The handleClick function will be triggered when the button is clicked, displaying the text of the div inside the black card.
- To access the div element, we will import the useRef Hook.
- Then, create a ref and pass it to the DOM element div as the ref attribute.
- useRef returns an object having a property, i.e., current. For accessing the div element on which we set the ref prop, we need to access the current property on the ref object.
- When we pass a ref prop to an element, <div ref={ref} />, React sets the .current property of the ref object to the corresponding DOM node.
- By accessing the style property of the element, we can get an object containing the element’s styles.
- React will put a reference to the DOM element into the ref. current, which we can access through an event handler handleClick.
- We will change the style of the div as ref.current.style.backgroundColor=’ black’; followed by other styles.
File name: App.js
Javascript
|
Step 7: Your index.js file should look like this. The index.js file serves as the main entry point, and inside it the App.js file is rendered at the root ID of the DOM.
File name: index.js
Javascript
|
Step to run the application: Run the application by using the following command:
npm start
Output: By default, the React project will run on port 3000. You can access it at localhost:3000 on your browser. By clicking the button, we can see how the useRef hook has changed the style of the div.
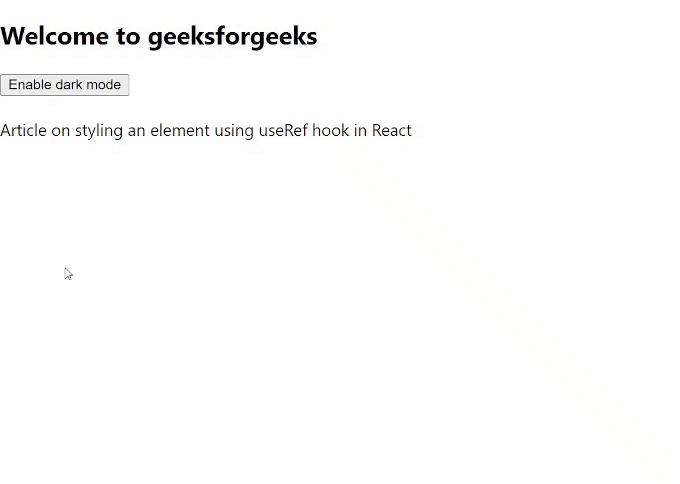
Output: Changing styles of an element using the useRef hook
In this article, we will learn how to change an element’s style using the useRef hook. UseRef() allows us to create a reference to a DOM element and keep track of variables without causing re-renders.
The useRef has a lot of importance in development, one of which is accessing the DOM directly. The useRef hook enables us to handle DOM manipulations. We can directly access DOM elements by adding a ref attribute to an element. By accessing the DOM, we can even manipulate the styles of an element. We can use the style property to set styles on the DOM element.
Syntax:
const refContainer = useRef(initialValue);
To use a ref to change an element’s style in React:
- We will import the useRef hook to access the DOM element we wish to style
- Then, declare a ref and pass it to the DOM element as the ref attribute.
- useRef returns a reference object having a single property, i.e., current.
- React puts a reference to the DOM element into the ref current, which we can access through an event handler.
- We will access the element via the current property on the ref.
- Finally, we will update the element’s style through ref.current.style.
Let us understand this concept through the following example.
Approach: We will create a div element with some text. Then we will use the useRef hook to change the style of this div. We will create a button that will enable dark mode, i.e., change the div’s background to black. Below is the step-by-step implementation.
Step 1: Make a project directory, head over to the terminal, and create a react app named “gfg-card” using the following command:
npx create-react-app gfg-card
Step 2: After the gfg-card app is created, switch to the new folder gfg-card using the following command:
cd gfg-card
Step 3: We will modify the folder and keep the files we need for this project. Make sure your file structure looks like this.
Project Structure: It will look like the following.
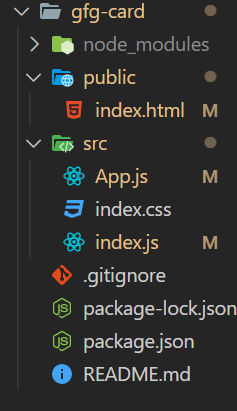
Final Directory Structure
Step 5: Add code to your index.html file. Include the following code in your index.html file, located in the public folder of your project directory.
File name: index.html
HTML
|
Step 6: Now change the style of the div element by using the useRef hook. In the App component, create a div element and a button to enable the dark mode for the div. We will add an event handler to the button. The handleClick function will be triggered when the button is clicked, displaying the text of the div inside the black card.
- To access the div element, we will import the useRef Hook.
- Then, create a ref and pass it to the DOM element div as the ref attribute.
- useRef returns an object having a property, i.e., current. For accessing the div element on which we set the ref prop, we need to access the current property on the ref object.
- When we pass a ref prop to an element, <div ref={ref} />, React sets the .current property of the ref object to the corresponding DOM node.
- By accessing the style property of the element, we can get an object containing the element’s styles.
- React will put a reference to the DOM element into the ref. current, which we can access through an event handler handleClick.
- We will change the style of the div as ref.current.style.backgroundColor=’ black’; followed by other styles.
File name: App.js
Javascript
|
Step 7: Your index.js file should look like this. The index.js file serves as the main entry point, and inside it the App.js file is rendered at the root ID of the DOM.
File name: index.js
Javascript
|
Step to run the application: Run the application by using the following command:
npm start
Output: By default, the React project will run on port 3000. You can access it at localhost:3000 on your browser. By clicking the button, we can see how the useRef hook has changed the style of the div.
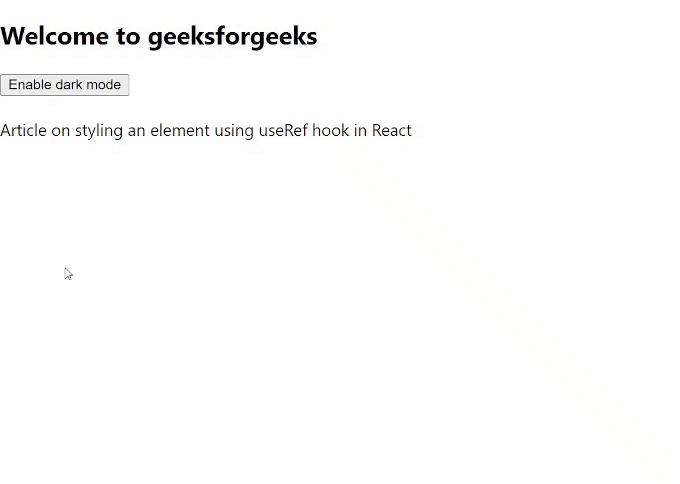
Output: Changing styles of an element using the useRef hook